Backend Engineering With Go
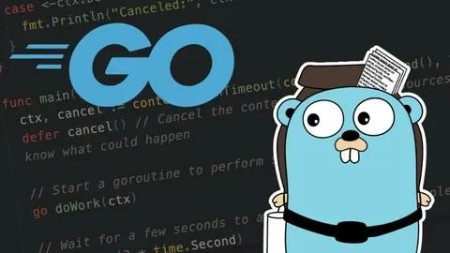
Backend Engineering With Go
Published 9/2024
MP4 | Video: h264, 1920x1080 | Audio: AAC, 44.1 KHz
Language: English | Size: 11.24 GB | Duration: 15h 7m
Full Guide to Building Production Backend Web Applications with Go, PostgreSQL, Docker and Deploying to the Cloud
What you'll learn
Learn the Fundamentals of Web Development with Go
Build Production Grade Applications with Go
Learn to Weight and Think about Software Design Decisions
Learn to Build and Deploy Go APIs to the Cloud
Learn How to Scale and manage Traffic
Learn How to Connect a Postgres Database with a Go Server
Requirements
Basic Go Knowledge
Description
In this project-based course, we will build a complete REST API in Go from scratch and deploy it to the cloud, ready to handle real traffic and scale affordably.Our journey begins by creating a simple TCP server, which will help us explore the net/http package in Go and understand the basics of handling network communication.Next, we'll dive into the theory behind building reliable and composable Go web applications. From there, we'll develop our project step-by-step, covering carefully curated topics such as request handling, middleware creation, database integration, request and database optimisations and rate limiting to equip you with the skills needed to ship real backend services to the cloud and manage real-world traffic efficiently.This course aims to provide you with the foundational knowledge required to build and understand backend systems, implement industry best practices, and create production-ready APIs that are secure, scalable, and maintainable. It is not just a step-by-step tutorial, but a comprehensive learning experience that prepares you for real-world scenarios.Legal Notice:All product and company names, logos, and trademarks featured on this thumbnail are the property of their respective owners. Their use in this course does not imply any affiliation, sponsorship, or endorsement by these companies.
Overview
Section 1: Introduction
Lecture 1 Project Overview
Lecture 2 Why Go with Go?
Lecture 3 Preface for Udemy Students
Lecture 4 Course Resources
Lecture 5 Getting your Tools Ready
Section 2: Project Architecture
Lecture 6 Design Principles for a REST API
Section 3: Building a server from TCP to HTTP
Lecture 7 TCP Server - net package
Lecture 8 Understanding Routing
Lecture 9 HTTP Server - The net/http package
Lecture 10 Encoding & Decoding JSON Requests
Section 4: Scaffolding our API Server
Lecture 11 Setting up your Development Environment
Lecture 12 Clean Layered Architecture
Lecture 13 Setting up the HTTP server and API
Lecture 14 Hot Reloading in Go
Lecture 15 Environment Variables
Section 5: Databases
Lecture 16 The Repository Pattern
Lecture 17 Implementing the Repository Pattern
Lecture 18 Persisting data with SQL
Lecture 19 Configuring the DB Connection Pool
Lecture 20 SQL Migrations
Section 6: Posts CRUD
Lecture 21 Marshalling JSON responses
Lecture 22 Creating a User Feed Post
Lecture 23 Getting a Post From a User
Lecture 24 Internal Errors Package
Lecture 25 HTTP Payload Validation
Lecture 26 DB Relationships & SQL Joins
Lecture 27 Adding Comments to Posts
Lecture 28 Updating and Deleting Posts
Lecture 29 Standardising JSON Responses
Lecture 30 Optimistic Concurrency Control
Lecture 31 Managing SQL Query Timeouts
Lecture 32 Database Seeding
Section 7: User Feed
Lecture 33 Creating the User Profile
Lecture 34 Adding Followers
Lecture 35 SQL Indexes
Lecture 36 User Feed Algorithm
Section 8: Filtering, Sorting, and Pagination
Lecture 37 Pagination and Sorting
Lecture 38 Feed Filtering
Section 9: Documentation
Lecture 39 Auto Generating Docs for the API
Lecture 40 Documenting the Handlers
Section 10: Structured Logging
Lecture 41 Adding a Logger
Section 11: User Creation
Lecture 42 User Registration Overview
Lecture 43 SQL Transactions
Lecture 44 User Activation
Section 12: Sending Emails
Lecture 45 Sending the Invitation Email
Lecture 46 Improving Further the Email
Lecture 47 Extra: Building the Confirmation UI
Section 13: Authentication
Lecture 48 Authentication Overview
Lecture 49 Basic Authentication
Lecture 50 Generating Tokens
Lecture 51 Validating Tokens
Section 14: Authorization
Lecture 52 Authorization Overview
Lecture 53 Authorization Database Setup
Lecture 54 Role Precedence Middleware
Lecture 55 Fixing the User Invitation
Section 15: Redis Caching
Lecture 56 Designing for Performance
Lecture 57 Caching the User Profile
Lecture 58 Invalidating the Cache On Update
Section 16: Testing
Lecture 59 Testing Overview
Lecture 60 Testing the User Handler
Lecture 61 Spies
Section 17: Graceful Shutdown
Lecture 62 Graceful Server Shutdown
Section 18: Rate Limiting
Lecture 63 Rate Limiting our API
Section 19: Handling CORS
Lecture 64 Handling CORS errors
Section 20: Server Metrics
Lecture 65 Basic Server Metrics
Section 21: Automation (CI/CD)
Lecture 66 Continuous Integration
Lecture 67 Changelog
Section 22: Production Deployment
Lecture 68 Deploying to Google Cloud
Beginners and Intermediate Developers,Advanced Software Engineers from Another Stack
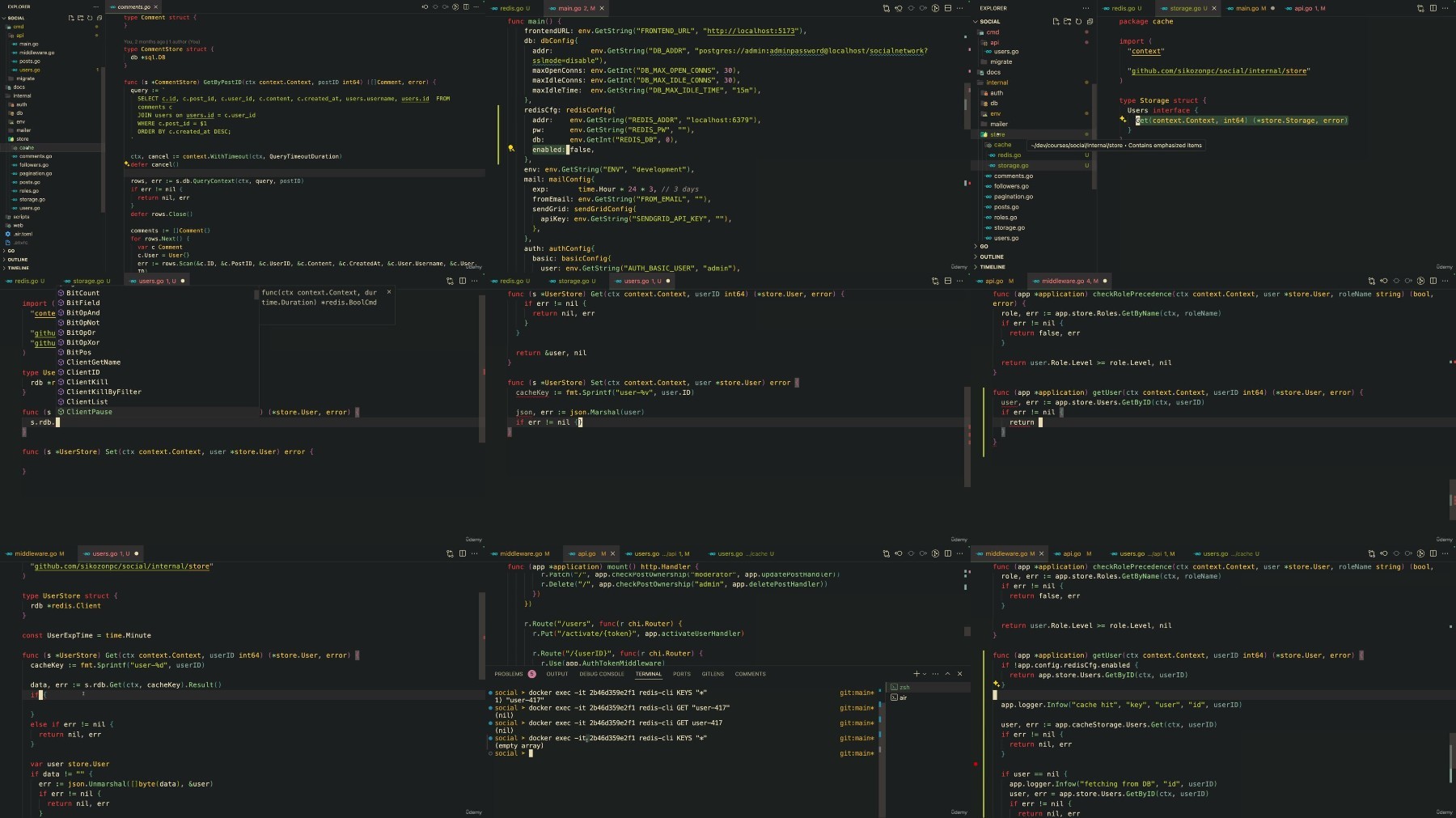
https://ddownload.com/k0bkngy0q7gj/Udemy_Backend_Engineering_with_Go_2024-9.part1.rar
https://ddownload.com/38jh5hf9y2x5/Udemy_Backend_Engineering_with_Go_2024-9.part2.rar
https://ddownload.com/4j748ck8g22s/Udemy_Backend_Engineering_with_Go_2024-9.part3.rar
https://ddownload.com/80u1jtux1gah/Udemy_Backend_Engineering_with_Go_2024-9.part4.rar
https://rapidgator.net/file/873c17d1adcfc96dcf29e671212a3947/Udemy_Backend_Engineering_with_Go_2024-9.part1.rar
https://rapidgator.net/file/e441f6a4082635c71b404f0861c09681/Udemy_Backend_Engineering_with_Go_2024-9.part2.rar
https://rapidgator.net/file/ec8642b88685cf8fbf64ee5a21aef86d/Udemy_Backend_Engineering_with_Go_2024-9.part3.rar
https://rapidgator.net/file/c21c60d750015add390a3cc27b223531/Udemy_Backend_Engineering_with_Go_2024-9.part4.rar
https://turbobit.net/r98yuv9hhbqt/Udemy_Backend_Engineering_with_Go_2024-9.part1.rar.html
https://turbobit.net/0wa5dhn90ohs/Udemy_Backend_Engineering_with_Go_2024-9.part2.rar.html
https://turbobit.net/xvpb52x4c0dj/Udemy_Backend_Engineering_with_Go_2024-9.part3.rar.html
https://turbobit.net/voc7ucw367pz/Udemy_Backend_Engineering_with_Go_2024-9.part4.rar.html
What you'll learn
Learn the Fundamentals of Web Development with Go
Build Production Grade Applications with Go
Learn to Weight and Think about Software Design Decisions
Learn to Build and Deploy Go APIs to the Cloud
Learn How to Scale and manage Traffic
Learn How to Connect a Postgres Database with a Go Server
Requirements
Basic Go Knowledge
Description
In this project-based course, we will build a complete REST API in Go from scratch and deploy it to the cloud, ready to handle real traffic and scale affordably.Our journey begins by creating a simple TCP server, which will help us explore the net/http package in Go and understand the basics of handling network communication.Next, we'll dive into the theory behind building reliable and composable Go web applications. From there, we'll develop our project step-by-step, covering carefully curated topics such as request handling, middleware creation, database integration, request and database optimisations and rate limiting to equip you with the skills needed to ship real backend services to the cloud and manage real-world traffic efficiently.This course aims to provide you with the foundational knowledge required to build and understand backend systems, implement industry best practices, and create production-ready APIs that are secure, scalable, and maintainable. It is not just a step-by-step tutorial, but a comprehensive learning experience that prepares you for real-world scenarios.Legal Notice:All product and company names, logos, and trademarks featured on this thumbnail are the property of their respective owners. Their use in this course does not imply any affiliation, sponsorship, or endorsement by these companies.
Overview
Section 1: Introduction
Lecture 1 Project Overview
Lecture 2 Why Go with Go?
Lecture 3 Preface for Udemy Students
Lecture 4 Course Resources
Lecture 5 Getting your Tools Ready
Section 2: Project Architecture
Lecture 6 Design Principles for a REST API
Section 3: Building a server from TCP to HTTP
Lecture 7 TCP Server - net package
Lecture 8 Understanding Routing
Lecture 9 HTTP Server - The net/http package
Lecture 10 Encoding & Decoding JSON Requests
Section 4: Scaffolding our API Server
Lecture 11 Setting up your Development Environment
Lecture 12 Clean Layered Architecture
Lecture 13 Setting up the HTTP server and API
Lecture 14 Hot Reloading in Go
Lecture 15 Environment Variables
Section 5: Databases
Lecture 16 The Repository Pattern
Lecture 17 Implementing the Repository Pattern
Lecture 18 Persisting data with SQL
Lecture 19 Configuring the DB Connection Pool
Lecture 20 SQL Migrations
Section 6: Posts CRUD
Lecture 21 Marshalling JSON responses
Lecture 22 Creating a User Feed Post
Lecture 23 Getting a Post From a User
Lecture 24 Internal Errors Package
Lecture 25 HTTP Payload Validation
Lecture 26 DB Relationships & SQL Joins
Lecture 27 Adding Comments to Posts
Lecture 28 Updating and Deleting Posts
Lecture 29 Standardising JSON Responses
Lecture 30 Optimistic Concurrency Control
Lecture 31 Managing SQL Query Timeouts
Lecture 32 Database Seeding
Section 7: User Feed
Lecture 33 Creating the User Profile
Lecture 34 Adding Followers
Lecture 35 SQL Indexes
Lecture 36 User Feed Algorithm
Section 8: Filtering, Sorting, and Pagination
Lecture 37 Pagination and Sorting
Lecture 38 Feed Filtering
Section 9: Documentation
Lecture 39 Auto Generating Docs for the API
Lecture 40 Documenting the Handlers
Section 10: Structured Logging
Lecture 41 Adding a Logger
Section 11: User Creation
Lecture 42 User Registration Overview
Lecture 43 SQL Transactions
Lecture 44 User Activation
Section 12: Sending Emails
Lecture 45 Sending the Invitation Email
Lecture 46 Improving Further the Email
Lecture 47 Extra: Building the Confirmation UI
Section 13: Authentication
Lecture 48 Authentication Overview
Lecture 49 Basic Authentication
Lecture 50 Generating Tokens
Lecture 51 Validating Tokens
Section 14: Authorization
Lecture 52 Authorization Overview
Lecture 53 Authorization Database Setup
Lecture 54 Role Precedence Middleware
Lecture 55 Fixing the User Invitation
Section 15: Redis Caching
Lecture 56 Designing for Performance
Lecture 57 Caching the User Profile
Lecture 58 Invalidating the Cache On Update
Section 16: Testing
Lecture 59 Testing Overview
Lecture 60 Testing the User Handler
Lecture 61 Spies
Section 17: Graceful Shutdown
Lecture 62 Graceful Server Shutdown
Section 18: Rate Limiting
Lecture 63 Rate Limiting our API
Section 19: Handling CORS
Lecture 64 Handling CORS errors
Section 20: Server Metrics
Lecture 65 Basic Server Metrics
Section 21: Automation (CI/CD)
Lecture 66 Continuous Integration
Lecture 67 Changelog
Section 22: Production Deployment
Lecture 68 Deploying to Google Cloud
Beginners and Intermediate Developers,Advanced Software Engineers from Another Stack
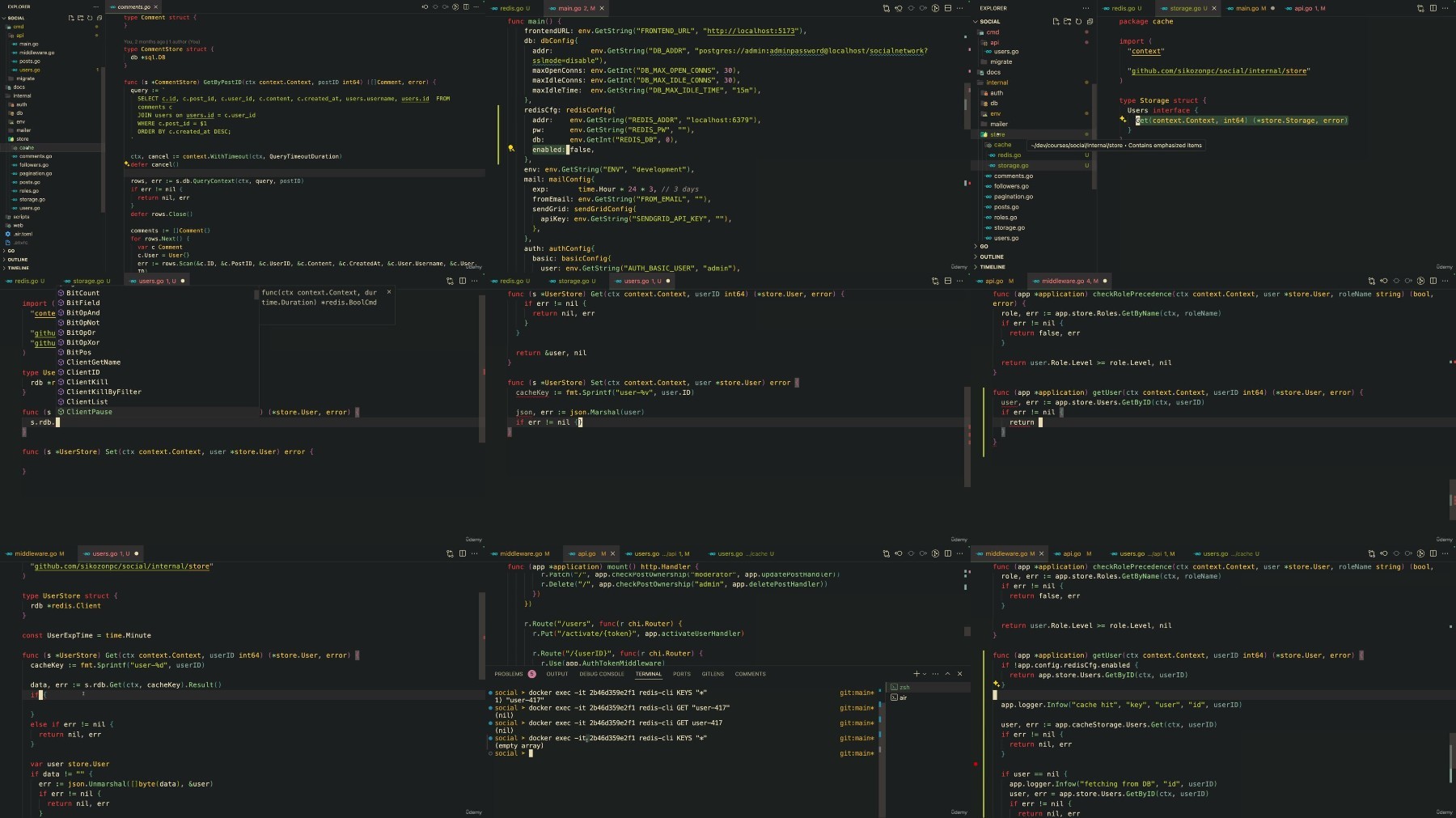
https://ddownload.com/k0bkngy0q7gj/Udemy_Backend_Engineering_with_Go_2024-9.part1.rar
https://ddownload.com/38jh5hf9y2x5/Udemy_Backend_Engineering_with_Go_2024-9.part2.rar
https://ddownload.com/4j748ck8g22s/Udemy_Backend_Engineering_with_Go_2024-9.part3.rar
https://ddownload.com/80u1jtux1gah/Udemy_Backend_Engineering_with_Go_2024-9.part4.rar
https://rapidgator.net/file/873c17d1adcfc96dcf29e671212a3947/Udemy_Backend_Engineering_with_Go_2024-9.part1.rar
https://rapidgator.net/file/e441f6a4082635c71b404f0861c09681/Udemy_Backend_Engineering_with_Go_2024-9.part2.rar
https://rapidgator.net/file/ec8642b88685cf8fbf64ee5a21aef86d/Udemy_Backend_Engineering_with_Go_2024-9.part3.rar
https://rapidgator.net/file/c21c60d750015add390a3cc27b223531/Udemy_Backend_Engineering_with_Go_2024-9.part4.rar
https://turbobit.net/r98yuv9hhbqt/Udemy_Backend_Engineering_with_Go_2024-9.part1.rar.html
https://turbobit.net/0wa5dhn90ohs/Udemy_Backend_Engineering_with_Go_2024-9.part2.rar.html
https://turbobit.net/xvpb52x4c0dj/Udemy_Backend_Engineering_with_Go_2024-9.part3.rar.html
https://turbobit.net/voc7ucw367pz/Udemy_Backend_Engineering_with_Go_2024-9.part4.rar.html
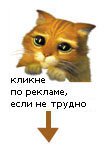