Java Collections Framework Core, Advanced & Interview Prep
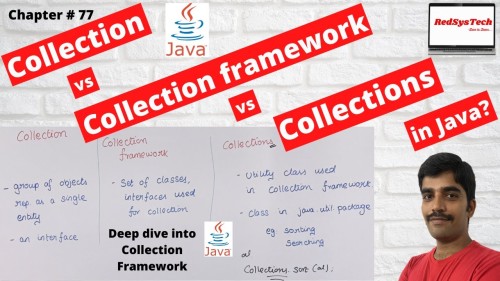
Java Collections Framework: Core, Advanced & Interview Prep
Published 11/2024
Created by Selfcode Academy
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz, 2 Ch
Genre: eLearning | Language: English | Duration: 75 Lectures ( 18h 43m ) | Size: 11.6 GB
Master Java collections, advanced concepts, Stream API, and interview prepration with practical examples and challenges.
What you'll learn
Students will understand the core concepts and structure of the Java Collections Framework.
Students will learn to implement core Java collection interfaces like List, Set, and Map.
Students will learn to apply Java 8 Stream API and lambda expressions to optimize collection operations.
Students will gain hands-on experience with concurrent collections and thread-safe operations.
Requirements
Basic knowledge of Java programming language.
Familiarity with object-oriented programming concepts.
No prior experience with collections is required; you'll learn everything needed.
Description
Unlock the power of the Java Collections Framework with this in-depth course designed to help you master Java's most important data structures and algorithms. Whether you are preparing for a technical interview, looking to improve your coding skills, or aiming to optimize your Java applications, this course will provide the knowledge and hands-on experience needed to excel.Module 1: Introduction to CollectionsLay a solid foundation by understanding the core concepts of Java collections, making it easier to implement more advanced topics later.Understand the Collection Framework and learn how it can enhance your Java programming efficiency.Get familiar with Map collections, arrays, and the benefits of using generics, which will help you write safer, more efficient code.Module 2: Core Interfaces of the Collection FrameworkMaster Java's core interfaces such as List, Set, Queue, and Map, which are key to handling data effectively in real-world applications.Learn about essential methods like Iterator, forEach(), and spliterator(), and how they enable better data traversal.Gain a strong understanding of List and Set interfaces, so you can choose the best data structure for your applications.Module 3: Collection Classes and ImplementationsUnderstand how to implement collections using ArrayList, LinkedList, HashSet, TreeSet, and others, and know when to use each for maximum performance.Learn thread-safe collections like Vector and Stack for scenarios where multi-threading is necessary.Develop an intuition for choosing the right collection class based on your application's requirements, optimizing both speed and memory usage.Module 4: Advanced ConceptsMaster advanced topics like synchronized collections, immutable collections, and performance considerations, which are crucial for building high-performance applications.Learn how to effectively use Iterator vs ListIterator to handle data iteration more efficiently.Gain insights into concurrent collections, preparing you for modern, multi-threaded application development.Module 5: Java 8 Enhancements to Collections & Stream APILearn how Java 8's Stream API transforms data manipulation by simplifying your code with lambda expressions and parallel streams.Explore advanced operations like collectors, internal iteration, and functional programming in Java, allowing you to write cleaner and more efficient code.Boost your productivity by mastering the Stream API, which is widely used in modern Java applications.Module 6: Concurrent CollectionsMaster concurrent collections, a must-have skill for developing scalable, thread-safe applications that perform well under high load.Learn about key collections like ConcurrentHashMap, CopyOnWriteArrayList, and BlockingQueue, which will empower you to handle concurrency challenges in your projects.Gain practical knowledge to write multi-threaded code that works efficiently in a real-world environment.Module 7: Algorithms and Utilities in CollectionsLearn essential algorithms like sorting, searching, and shifting to optimize data handling in your applications.Understand how to use utility methods for common tasks, which will save you time and effort when working with collections.Enhance your problem-solving skills by mastering useful operations like reversing, rotating, and filling, which are crucial for manipulating large data sets efficiently.Module 8: Practical Examples and Use CasesApply your knowledge with real-world projects such as managing a to-do list, implementing caching mechanisms, and task scheduling using collections.Develop practical skills that directly translate to job-ready expertise by working with collections to solve everyday software development challenges.Get valuable experience in building systems that handle real-world data management needs.Module 9: Collection Framework in InterviewsPrepare for technical interviews with a strong focus on Java collections. Understand common interview questions and practice real coding challenges to boost your confidence.Learn how to answer tricky interview questions like:How does ConcurrentHashMap ensure thread-safety?What's the difference between HashMap and LinkedHashMap?How does TreeMap handle sorting?Tackle HackerRank challenges to solidify your skills and prepare for interviews with confidence.By completing this course, you will:Master Java collections and confidently apply them to solve real-world problems.Gain a deep understanding of both core and advanced collection concepts, enhancing your ability to design efficient, optimized applications.Be prepared for technical interviews with Java collections, improving your chances of securing a job in software development.Improve the performance and scalability of your Java applications by leveraging powerful collection frameworks and concurrency tools.
Who this course is for
Java developers looking to deepen their understanding of the Collections Framework.
Intermediate Java learners aiming to enhance their skills for technical interviews.
Professionals seeking to master concurrent collections and Java 8 features for modern applications.
Homepage
https://rapidgator.net/file/56bb866f3ea0dcbb4c1d5a0788716105/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part13.rar.html,_Advanced_&_Interview_Prep.part13.rar.html
https://rapidgator.net/file/73582b7a294283f76a3d2eb688c4e2f8/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part12.rar.html,_Advanced_&_Interview_Prep.part12.rar.html
https://rapidgator.net/file/9b0c17e53e42fa1893008a2ea860540b/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part11.rar.html,_Advanced_&_Interview_Prep.part11.rar.html
https://rapidgator.net/file/6cc79e3ceef82fb607ed3a2ee9caef65/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part10.rar.html,_Advanced_&_Interview_Prep.part10.rar.html
https://rapidgator.net/file/31d8dc46e488010d72a0c2f3df49ca1d/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part09.rar.html,_Advanced_&_Interview_Prep.part09.rar.html
https://rapidgator.net/file/994ca95a9b0991f1c6de178bbe6d7eb9/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part08.rar.html,_Advanced_&_Interview_Prep.part08.rar.html
https://rapidgator.net/file/c98103b261895569022d37e946c977c4/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part07.rar.html,_Advanced_&_Interview_Prep.part07.rar.html
https://rapidgator.net/file/6f994635a17a7484255a3e20640816bf/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part06.rar.html,_Advanced_&_Interview_Prep.part06.rar.html
https://rapidgator.net/file/264b2a2760a0a2dda3e9f279ce921731/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part05.rar.html,_Advanced_&_Interview_Prep.part05.rar.html
https://rapidgator.net/file/c2991813fce47b3d87ef55afc5b23123/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part04.rar.html,_Advanced_&_Interview_Prep.part04.rar.html
https://rapidgator.net/file/ecd0dd6d857aa0df40e988e1f56e0e8e/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part03.rar.html,_Advanced_&_Interview_Prep.part03.rar.html
https://rapidgator.net/file/376101fb348b89e280f01a3cc423c758/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part02.rar.html,_Advanced_&_Interview_Prep.part02.rar.html
https://rapidgator.net/file/d96802a5d3400379302fea8710d4aaec/Java_Collections_Framework_Core,_Advanced_&_Interview_Prep.part01.rar.html,_Advanced_&_Interview_Prep.part01.rar.html
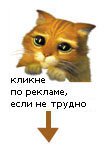